Getting Started with Tex Brodus
Alright, let’s talk about this thing I ended up calling ‘tex brodus’. It wasn’t some grand plan, you know? It just sort of… happened. I was wrestling with a project a while back, trying to get textures updated smoothly across a bunch of different displays. Sounds simple, right? Turned out to be a real headache.
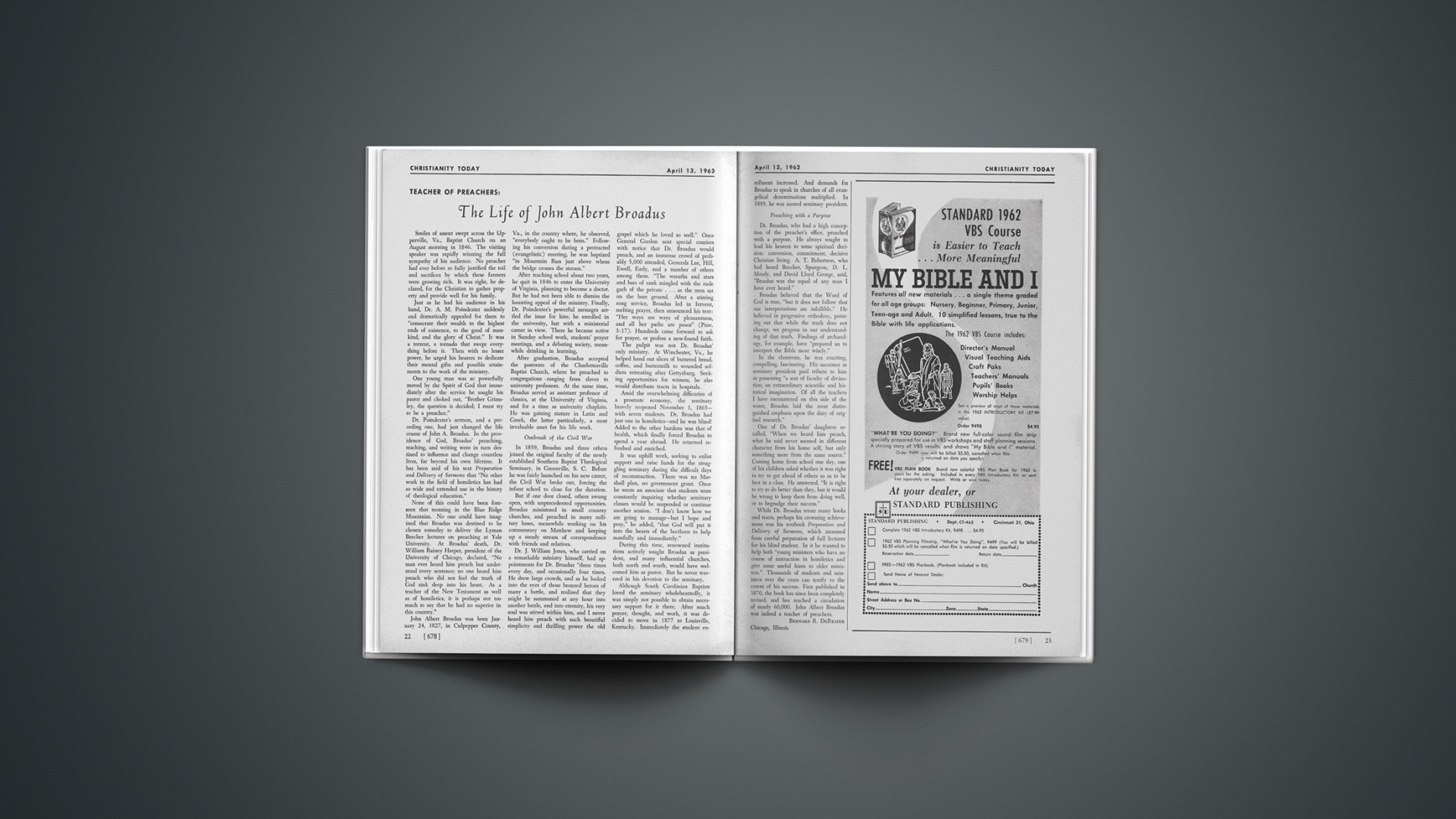
The setup was messy. We had multiple outputs, different resolutions, and the source textures were changing constantly. Trying to sync everything up manually or with the standard tools we had was just… slow. Painfully slow. We’d see tearing, lag, sometimes things just wouldn’t update at all. It was frustrating, honestly. Spent way too many late nights just staring at screens, trying to figure out why one display was showing something completely different from another.
Digging In and Making a Mess
So, I decided I had to get my hands dirty. First, I tried tweaking the existing pipeline. Played around with buffering strategies, tried different compression formats, messed with network protocols. Some things helped a little, but nothing really fixed the core problem. It felt like putting band-aids on a leaky pipe.
Here’s what I ran into mostly:
- Timing Issues: Getting updates to all displays at roughly the same time was a nightmare.
- Performance Hits: Pushing high-res textures around constantly bogged down the system.
- Complexity: The code managing all this was becoming a tangled mess. Hard to debug, harder to maintain.
After banging my head against the wall for a bit, I thought, “Okay, maybe I need a different approach.” I started thinking about just broadcasting the essential texture data efficiently, rather than managing complex state across all endpoints. That’s where the idea for ‘tex brodus’ started forming. It wasn’t a fancy name, just short for ‘texture broadcasting’, basically.
Building the Thing
I began by stripping things down. Focused on getting the raw texture data pushed out reliably from the source. Spent a good chunk of time writing some simple network code, trying to keep it lightweight. Didn’t use any fancy libraries at first, just basic socket stuff to see if the concept even worked.
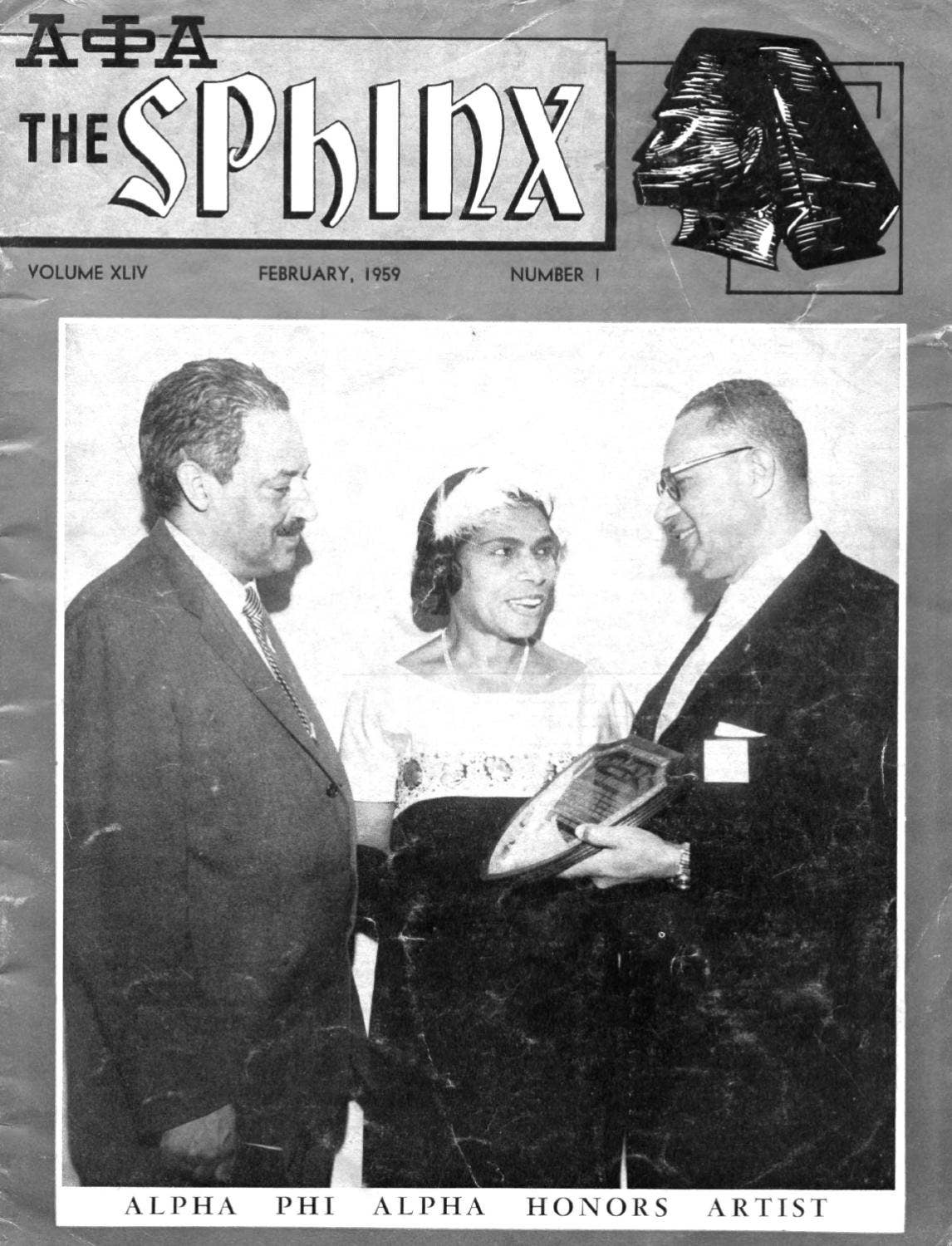
The core steps were something like this:
I set up a central point where the texture updates originated. Made sure it could grab the latest texture quickly.
Then, I worked on the ‘broadcast’ part. Figured out a simple protocol – nothing complex, just enough to say “here’s a new texture chunk” and send it out to whoever was listening.
On the receiving end, the clients just listened for these broadcasts. When new data came in, they pieced the texture back together and slapped it onto the display. Kept the client logic super simple.
It wasn’t perfect straight away, obviously. Had plenty of issues with dropped packets, ordering problems, and making sure the clients could keep up. Lots of trial and error. Lots of `print()` statements everywhere to see what was going on. It felt more like plumbing than elegant engineering some days.
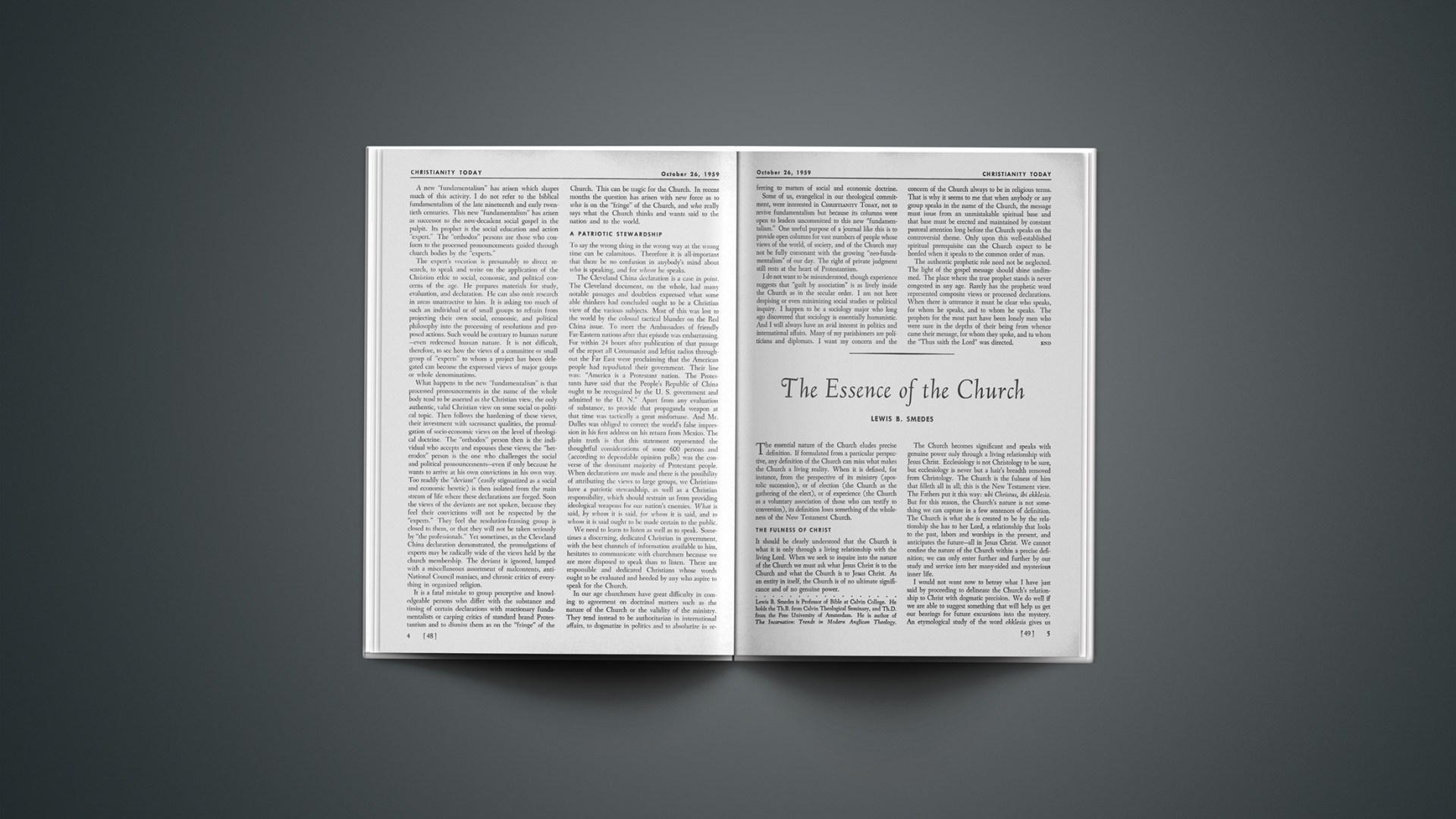
Did it Work?
Yeah, surprisingly, it did. Long story short, after a lot of tweaking and coffee, ‘tex brodus’ actually started doing its job. The updates became much smoother. The lag was significantly reduced. It wasn’t hitting the system performance nearly as hard as the old methods.
The main win was simplicity. By focusing on just getting the data out there reliably, we bypassed a lot of the complex synchronization logic that was causing headaches before. The receiving clients were dumb and happy, just taking data as it came.
It wasn’t a universal solution, mind you. Had its own quirks. If the network got really congested, things could still get delayed. And it was pretty specific to our particular problem – probably wouldn’t work everywhere. But for what we needed? It got the job done. Felt good to build something, even something rough around the edges, that actually solved a real problem we were facing day-to-day. Sometimes the simple, brute-force approach is the way to go.