Alright, folks, gather ’round! Let me tell you about this little project I just wrapped up. I’m calling it “six times lucky” because, well, it took me six tries to get it right!
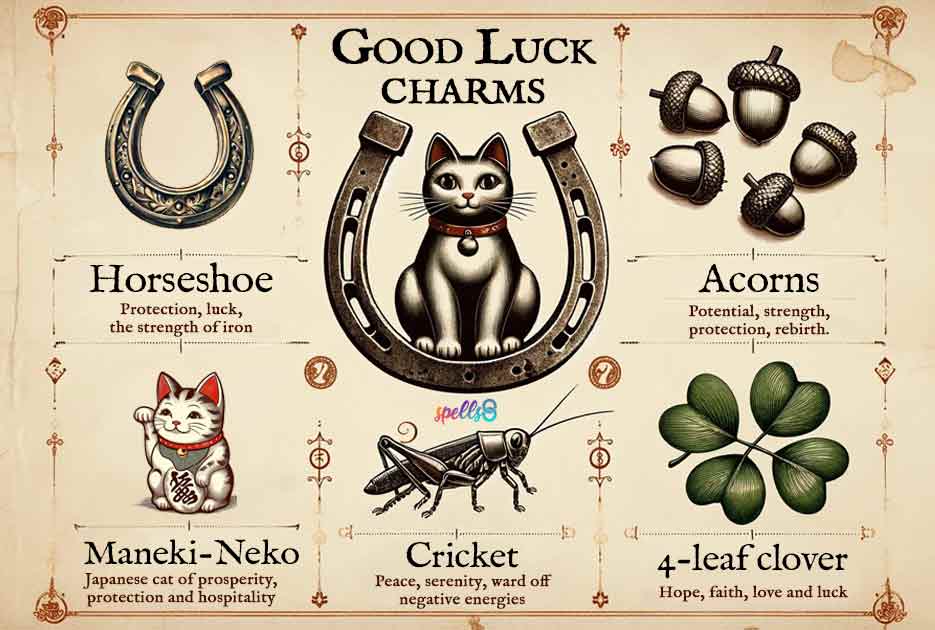
So, the idea was simple enough: I wanted to create a script that would automatically rename a bunch of files according to a specific pattern. I had this folder full of images, all named like “image_*”, “image_*”, and so on. What I wanted was to rename them to include a prefix based on the date they were taken, something like “20240101_image_*”.
Attempt #1: The Naive Approach
I jumped right in with a simple Python script using the os
module. I looped through the files, tried to extract the date from the image’s EXIF data using the PIL
library, and then rename the file. Sounded easy, right? Wrong! Turns out, not all images have EXIF data, and some have it in different formats. My script choked on the first image without EXIF and threw a big, ugly error. Fail!
Attempt #2: Handling Exceptions (or Trying To)
Okay, I thought, I’ll just add some error handling. I wrapped the EXIF extraction part in a try...except
block. If it failed, I’d just skip the image and move on. But then I realized, what if the date is missing? I can’t just rename it without a date. So, I added a default date, something like “unknown_date”. It worked…sort of. Now my script didn’t crash, but I had a bunch of files named “unknown_date_image_*”. Not ideal.
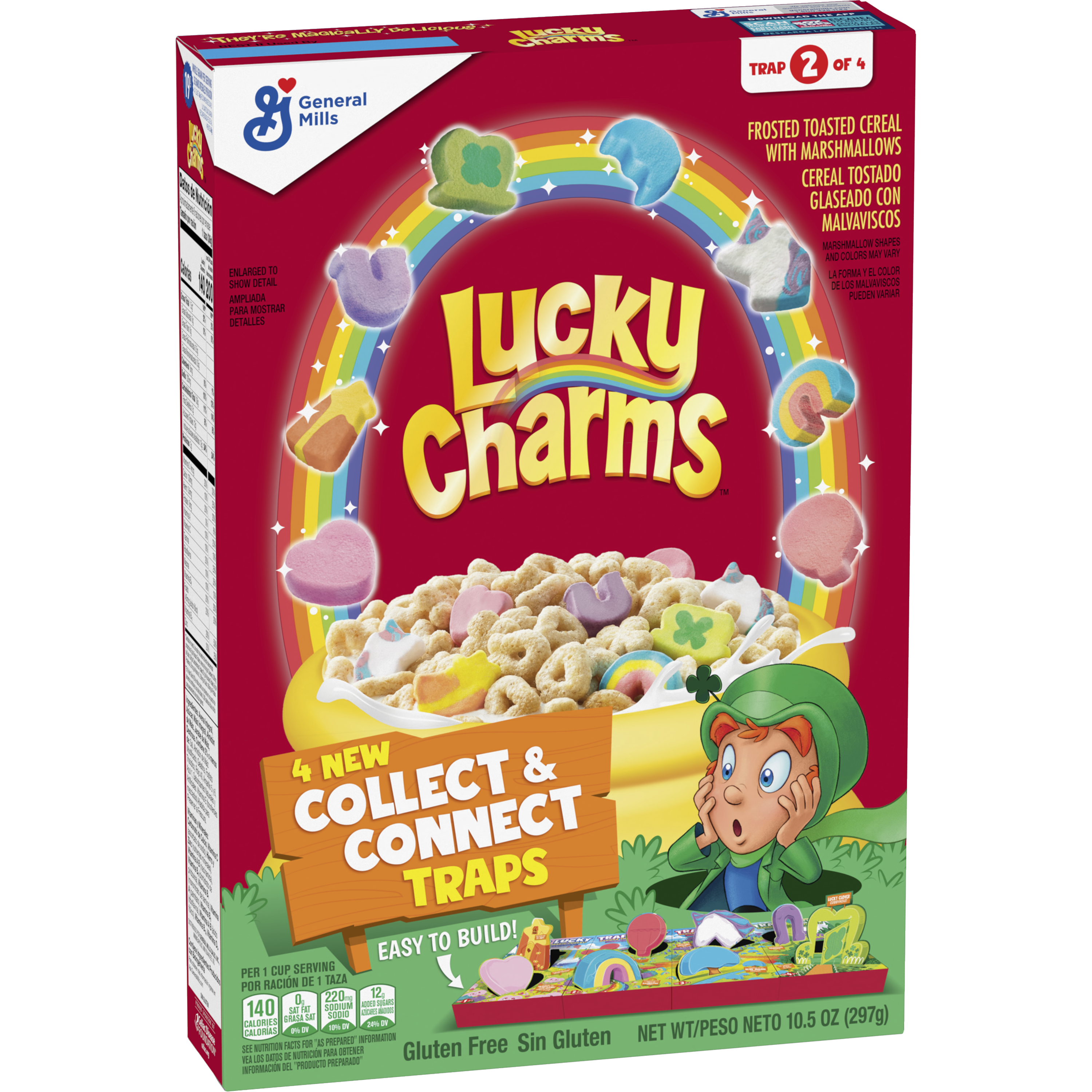
Attempt #3: Digging Deeper into EXIF
I decided to spend some more time figuring out the EXIF data. I found a different library, exifread
, that seemed to be more robust. I also learned that the date might be stored in different EXIF tags. I modified my script to check multiple tags for the date. This got me further, but I still ran into images with no date information. And some images had corrupted EXIF data that crashed the library. Argh!
Attempt #4: Default Date Strategy Revisited
Back to the drawing board. If I couldn’t reliably get the date from the EXIF data, I needed a better strategy for the default date. I thought, maybe I could use the file creation date as a fallback. I found some code to get the file creation date, but it turned out to be platform-dependent (worked on my Mac, but not on Windows). More complexity! I settled on using the last modification date as a compromise.
Attempt #5: Putting It All Together (Almost)
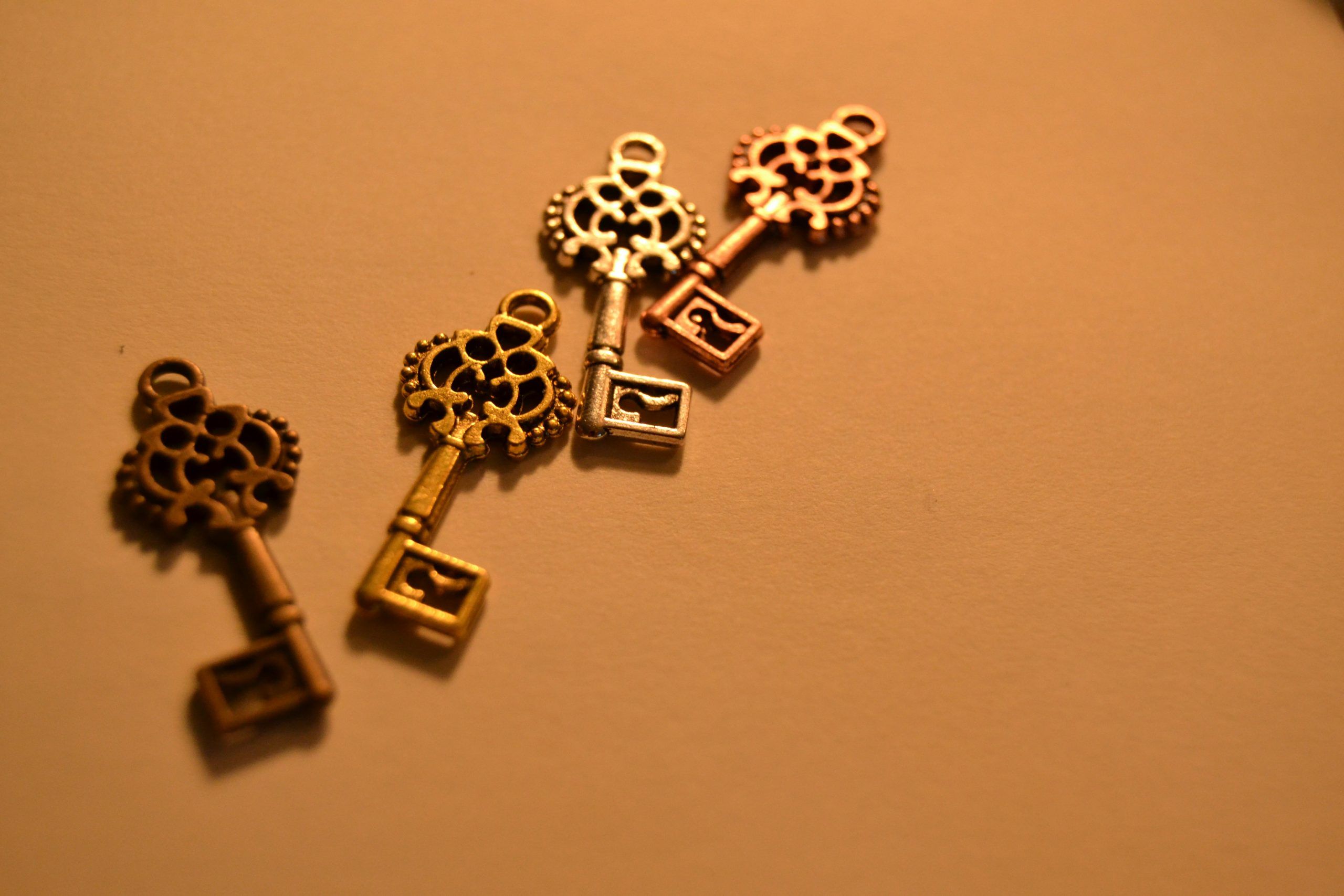
Okay, time to put it all together: try exifread
first, check multiple EXIF tags, handle errors gracefully, fall back to the last modification date if EXIF fails. I ran the script, and it worked! …for most of the files. Then I noticed that some of the renamed files had spaces in the date, like “2024 01 01_image_*”. This was because the EXIF date format was inconsistent. I added a step to clean up the date string before renaming.
Attempt #6: Success (Finally!)
Finally! After all that, I had a script that reliably renamed all my images. It handles missing EXIF data, inconsistent date formats, and platform differences. It’s not the prettiest code, but it gets the job done. I even added a command-line argument to specify the directory to process, so I can use it on different folders.
What I Learned
- Don’t underestimate the complexity of “simple” tasks.
- Error handling is crucial, especially when dealing with external data.
- There’s always more than one way to do something (and some ways are better than others).
- Sometimes, “good enough” is better than “perfect”.
So, there you have it: my “six times lucky” journey. It was frustrating at times, but I learned a lot along the way. And now I have a handy script that I can use again and again. Plus, a good story to tell!