Okay, here’s my attempt at a blog post based on your instructions, about a project called “colby pictures.” I’m aiming for that chatty, slightly rough-around-the-edges style.
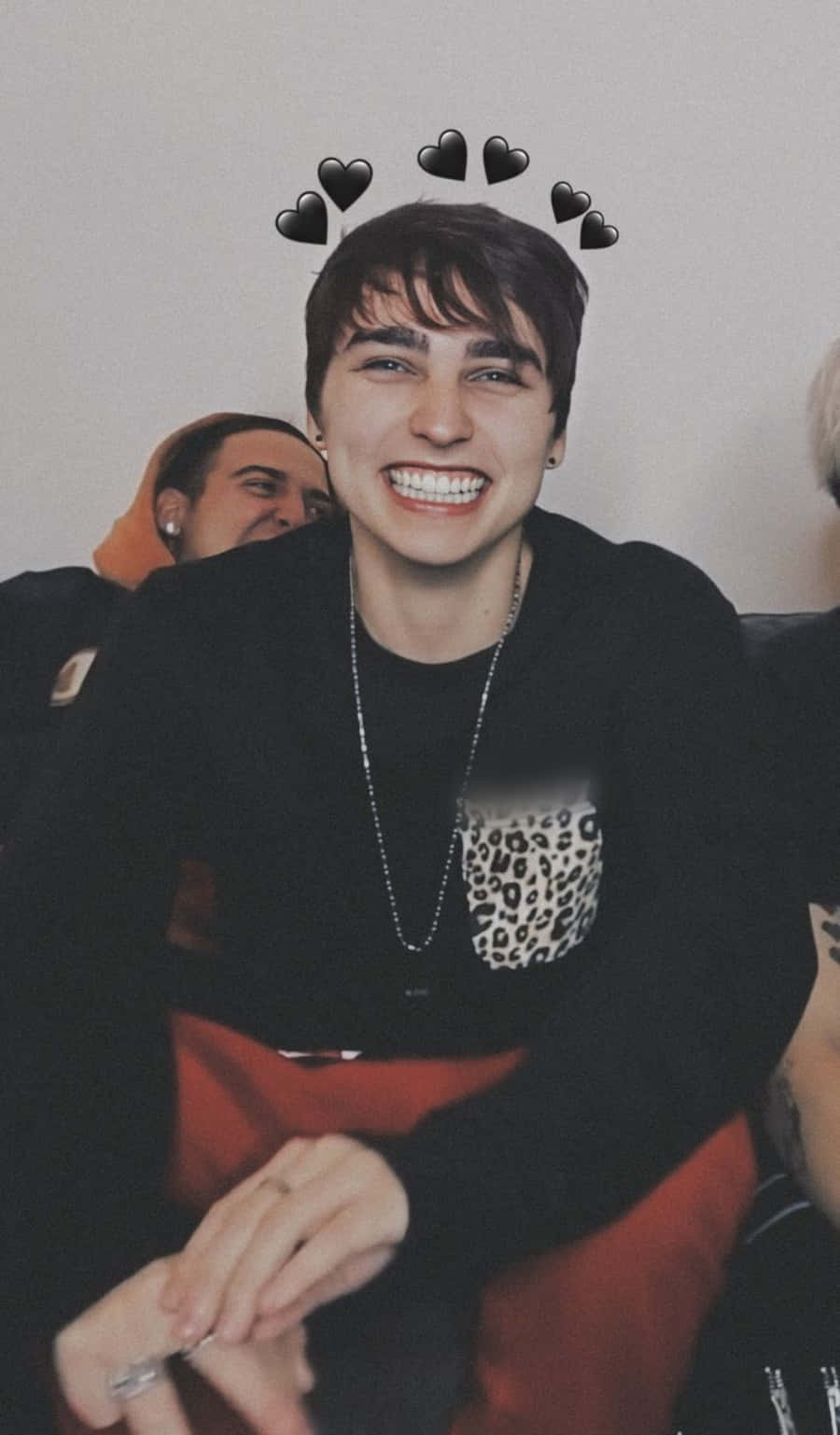
Alright folks, so I messed around with something I’m calling “colby pictures” recently, and thought I’d share how it went down. It’s not gonna be a super polished tutorial, just me rambling about what I did.
Basically, I wanted to see if I could throw together a little script that would grab images based on a search term and do some basic stuff with them. Like, resize ’em, maybe add a watermark, that kind of thing.
First things first, I needed to figure out how to actually get the pictures. I ended up using a search engine API. There are a bunch out there, some free, some paid. I went with one of the free-ish ones to start. They usually give you a certain number of requests per month before you gotta cough up the dough.
After signing up and getting my API key, I started to code. My language of choice was Python, cause it’s what I know. I installed the requests library, and started messing around with some basic API calls. Initially, just trying to get some JSON data back based on a simple search query. After fighting with the documentation and the API structure for about an hour, I finally got some image URLs to pop out.
Next up was actually downloading the images. This involved looping through the list of URLs I got from the API and using the requests
library again to fetch the image data. Then, I wrote the image data to a file. Nothing too fancy, just a basic open()
and write()
operation.
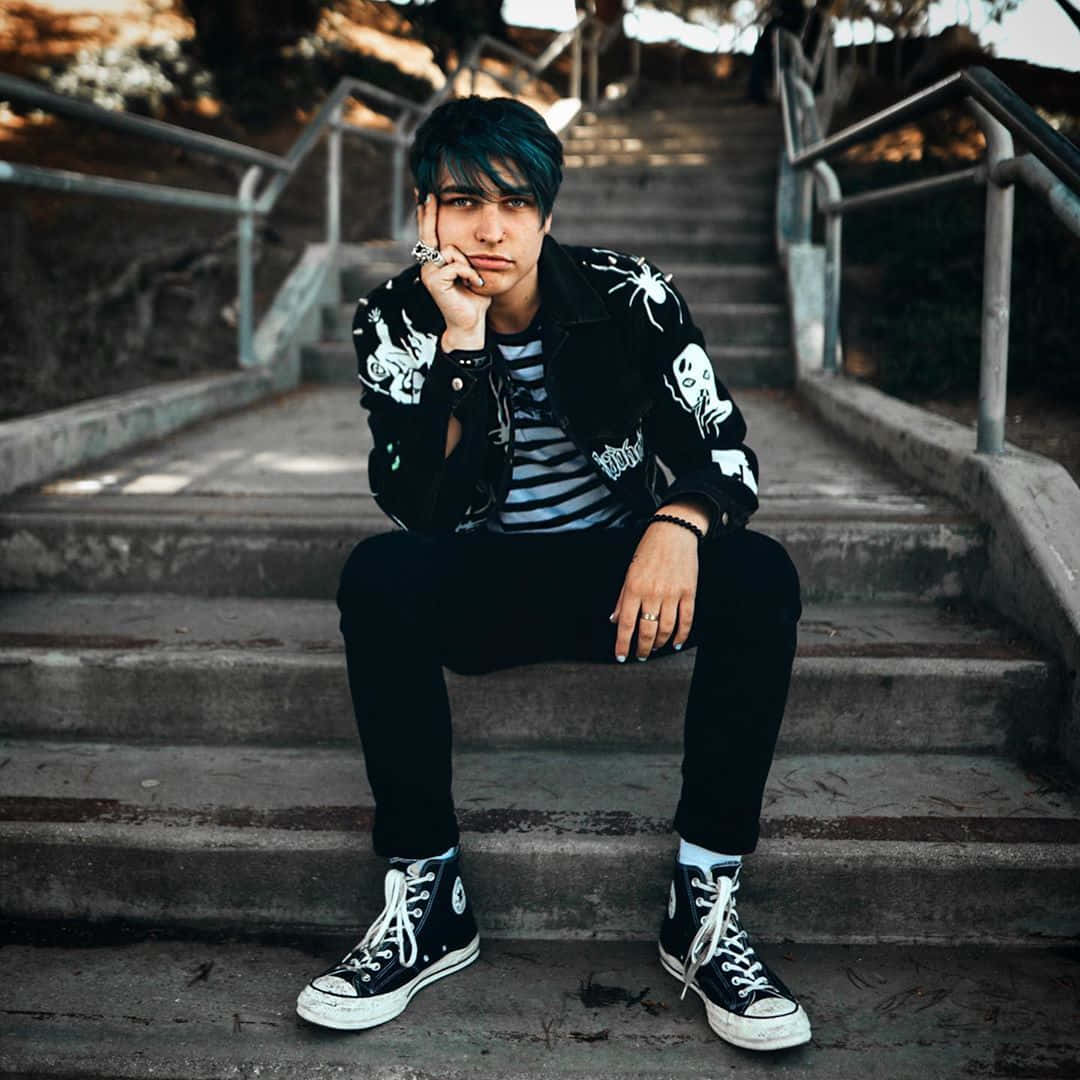
Okay, so now I had a pile of images sitting in a folder. Time to actually do something with them. I wanted to resize them, so I used Pillow (PIL) library. You gotta install it first: pip install Pillow
. Then, I could open each image with Pillow, calculate the new dimensions (I wanted to scale them down), and then save the resized image to a new folder.
Watermarking was next. Again, using Pillow, I opened the original image and a watermark image (a transparent PNG I created). Then, I calculated where to position the watermark (usually the bottom right corner), and pasted it onto the original image. Saved that out to another folder. It’s super basic, but it did the trick. The watermark wasn’t the most subtle thing ever, but hey, it’s a start.
So, the whole process looked something like this:
- Get API key from image search provider.
- Write Python script to:
- Make API request based on a search term.
- Parse the JSON response to extract image URLs.
- Download images from those URLs.
- Resize the downloaded images using Pillow.
- Add a watermark using Pillow.
- Save the processed images to a new directory.
- Run the script and watch it chug along.
It wasn’t perfect. Error handling was minimal (if a URL was broken, the script just crashed), and the API I was using had some limitations. But it worked! I had a folder full of resized, watermarked images based on my search term.
What I learned? APIs can be a pain in the butt at first. Pillow is super useful for image manipulation. And even a simple script like this can be a cool starting point for more complex image processing stuff.
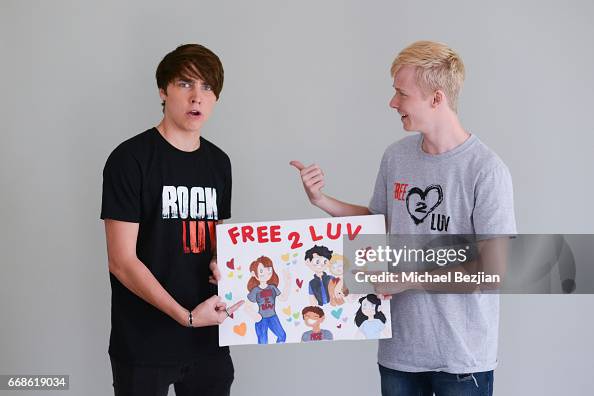
That’s pretty much it. “colby pictures” in a nutshell. Maybe I’ll clean it up and make it a real project someday. But for now, it was a fun little experiment.