Alright, so today I’m gonna walk you through this thing I was messing with called “green mile brutus.” It’s a bit of a clunky name, I know, but bear with me.
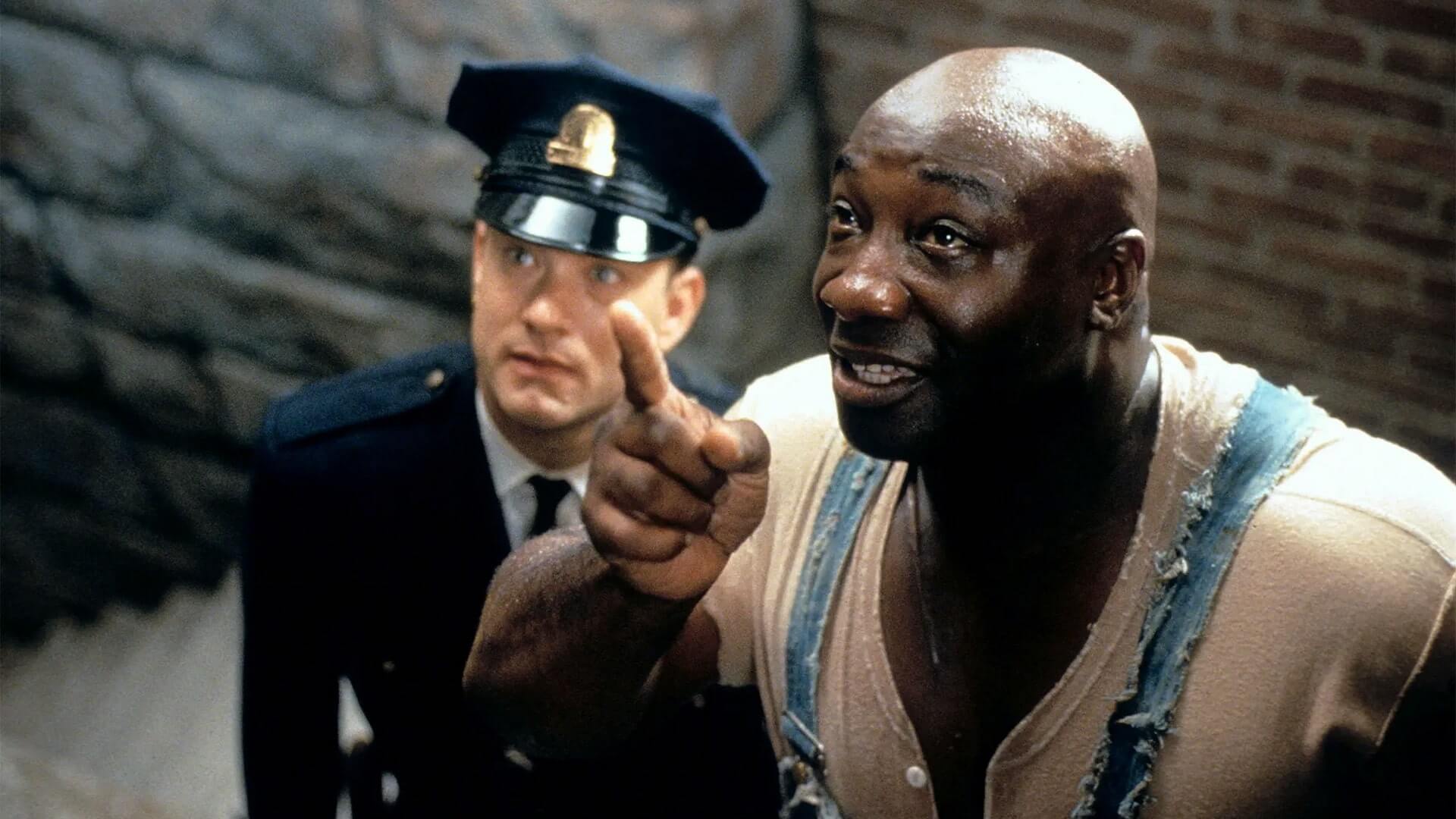
First off, I started with the basics. I had this idea floating around in my head, right? Something about data processing, but I needed a concrete problem to tackle. So, I grabbed this dataset – a real mess of different file formats and structures. Perfect!
I spent a good chunk of time just cleaning things up. I’m talking about writing Python scripts to convert CSV files to JSON, stripping out weird characters, normalizing date formats, the whole nine yards. It was tedious, but you gotta get your hands dirty sometimes, right?
Next up, I needed a place to stash all this cleaned data. I opted for a simple PostgreSQL database. I spun up a Docker container with Postgres, defined my schema – nothing too fancy, just enough to hold the data I was working with. After that, I wrote some more Python code to load the cleaned data into the database. It was a bit slow at first, so I spent some time optimizing the insertion queries. Turns out batch inserts are your friend!
Now, here comes the interesting part. I wanted to run some actual analysis. I started by writing some SQL queries directly in the Postgres console. Just basic stuff, like counting rows, calculating averages, and grouping data by different categories. But then, I wanted to get a bit fancier. I decided to hook up a Jupyter notebook to the database using the psycopg2
library.
With the Jupyter notebook, I could write more complex SQL queries, visualize the data using libraries like matplotlib
and seaborn
, and even build some simple machine learning models using scikit-learn
. I spent a few days just experimenting with different features, trying to see if I could uncover any hidden patterns or insights in the data. It was a lot of trial and error, but I learned a ton.
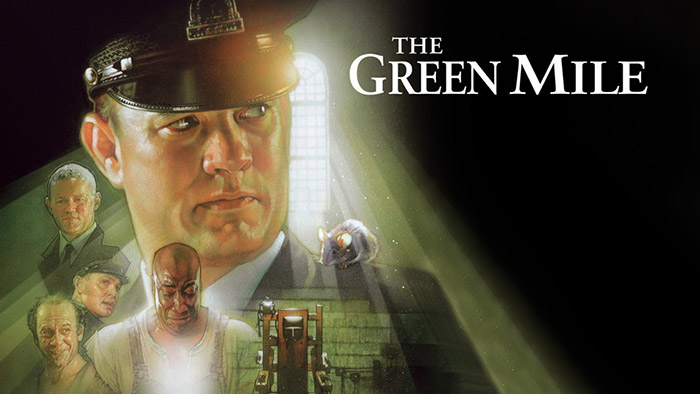
One challenge I ran into was with performance. Some of the queries were taking forever to run, especially when I was working with large subsets of the data. So, I had to do some performance tuning. I added indexes to the database tables, rewrote some of the SQL queries to be more efficient, and even experimented with different database configurations.
Finally, I wanted to make this whole thing reproducible. So, I wrapped everything up in a Docker Compose file. This allowed me to spin up the entire environment – the Postgres database, the Jupyter notebook – with a single command. I also created a Makefile with common tasks like cleaning the data, loading the data into the database, and running the analysis. This made it easy for anyone to reproduce my results, even if they didn’t have all the necessary software installed.
Lessons learned? Well, cleaning data is always more time-consuming than you think. Batch inserts are crucial for performance when loading data into a database. Jupyter notebooks are great for exploratory data analysis. And Docker Compose is your friend when it comes to reproducibility.
- Data cleaning takes time.
- Batch inserts boost database loading.
- Jupyter notebooks rock for exploration.
- Docker Compose ensures reproducibility.
It wasn’t perfect, and there’s still plenty of room for improvement. But hey, that’s the fun of it, right? Always something new to learn.